PHP Cheat Sheet
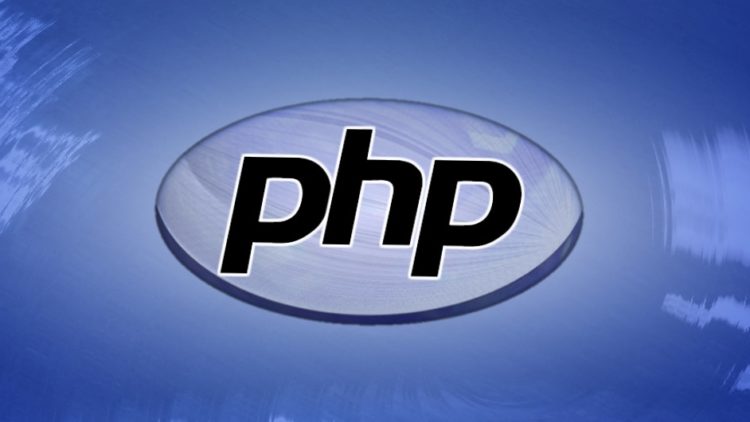
Contents
Code snippets of PHP that could be useful for someone(me)
Check password strength
Code stolen from: https://gist.github.com/bmcculley/9339529#file-password_strength-php
It is intended for this: Check for password strength, password should be at least n characters, contain at least one number, contain at least one lowercase letter, contain at least one uppercase letter, contain at least one special character.
<?php
$password_length = 8;
function password_strength($password) {
$returnVal = True;
if ( strlen($password) < $password_length ) {
$returnVal = False;
}
if ( !preg_match("#[0-9]+#", $password) ) {
$returnVal = False;
}
if ( !preg_match("#[a-z]+#", $password) ) {
$returnVal = False;
}
if ( !preg_match("#[A-Z]+#", $password) ) {
$returnVal = False;
}
if ( !preg_match("/[\'^£$%&*()}{@#~?><>,|=_+!-]/", $password) ) {
$returnVal = False;
}
return $returnVal;
}
?>